Google App Engine and Chrome, but mostly people, at #devfestau
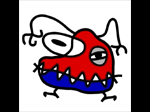
I have spent the last two days at Google DevFest 2010 in Sydney. The focus on Tuesday was Google App Engine and today we looked at Google Chrome, HTML5 and tools for the next generation of web applications. The talks were very interesting and I feel like I learnt something on both days, but the real value was in the people I met and the conversations I had.
Tuesday, 29th June - App Engine, Python and Scaling It
I have some web projects (including this blog), built on the LAMP stack and cobbled together in ways that would make a Scalability Engineer cringe. These sites work, but they won't scale and thanks to my fairly narrow demographic (Patrick O'brian fans and military history buffs), they haven't needed to thus far. However, over the last few months, this has started to change. My suite of iPhone applications have started to generate a respectable amount of traffic and I have had to start entertaining the idea of a more scalable backend architecture.
So this backend requirement, an embaressingly fervent love for Python, an interest in meeting Don Dodge in person and the gigabyte of bandwidth for free (yep, I am cheap) made the App Engine session a sensible choice.
Wednesday, 30th June - Chrome, HTML5 and the death knell of IE6
I have dabbled with Chrome extentions before and wanted to check out what was on the horizon with Chrome and HTML5.
I found the presentations on the new stuff coming in HTML5 compelling and frustrating at the same time. Basically this stuff is awesome and people are going to build some fantastic stuff with it, but until it is supported in a majority of browsers, I don't see how you can afford to spend much time on it. This is the nature of the business I suppose, but I am impatient, I want to use the new shiney stuff now!
I had a bit of fun playing around with javascript and SVG support in HTML5 during the coding labs at the end of the day. If you are using a modern browser you will see my logo blinking at you, if you aren't, then not so much. This is basically some Javascript accessing the DOM in an SVG file and messing with the properties:
People
Some of the people I met ...Pamela Fox

As usual, Pamela did a kick ass job. She's a great developer, very smart and genuinely passionate about building awesome software.
Don Dodge

I had seen Don Dodge interviewed on This Week in Startups and had followed his move from Microsoft to Google, so I was pretty keen to meet him in person. I thought his talk about how the web has evolved and is continuing to evolve was pretty insightful and, given his move from the heavyweight client world of Microsoft to Google and the cloud, I think this guy gets it.
I overcame my natural tendency to hide behind furniture and forced myself to step up and say hello. I found the man pretty approachable and had an amiable, if somewhat brief, conversation about how developers can benefit from the various cloud platforms and in particular how I can leverage it for mobile applications.
Alan Noble

I met Alan at the Mashup Australia hack-a-thon a few months ago, so I jumped at the chance to sit down with him and some other developers over lunch. Alan is the head engineer for Google Australia and had some interesting things to tell us about the day to day operation of Google from a developer perspective. I was particularly interested to hear about Australian Googler's involvement with the Cyber attacks on Google servers early this year. Alan described how Google security engineers are working around the clock (around the globe) on tracking and preventing cyber attacks. I wonder how many other companies were vicitims of the attacks that Google detected, but were completely unaware of it.
Patrick Chanezon

A very friendly French Googler in San Fransisco. This guy did an awesome presentation on App Engine for business and later showed how he fed his delicious bookmarks into the Google Prediction API to build personally meaningful tags for arbitary web sites. Very cool.
Halton Stewart

I met this guy towards the end of the day yesterday when he was having a chat with one of the Google guys about scaling the web apps that he had built. As far as I am concerned this guy is the poster boy for independant developers building scalable web sites on App Engine.
You might have seen advertisments on the web for a certain viral empire building game that has gained some notoriety for it's particular style of advertising. This notoriety probably contributed to a certain amount of self deprecation when Halton was describing what he had built. Basically he took a feature missing from the game and built a set of sites to satisfy that need. It turns out that 100s of thousands of other players wanted this feature too. His sites have done incredibly well and are generating real ad revenue every month. A couple of points:
- This is what being an awesome developer is all about. Halton had an itch and scratched it, built something awesome, that has real value for 100s of thousands of people.
- He started out using app engine, having never used Python or the platform before, and taught himself by 'doing'. Also, full of awesome!
- He encountered some nasty problems along the way (like the Python image encoder not supporting text) and hacked until it worked. Again, awesome.
- He made a choice to use App Engine rather than persisting with the familiar, but not so scalable LAMP stack, that he was used to. Outside the comfort zone, awesome!
So in summary, and with a little bit of re-iteration, this guy is crushing it and has absolutely nothing to be embarressed about. I was very impressed.
Geoff Mcqueen

Geoff gave a great presentation on Affinity Live and their experiences integrating Google Apps. Had an intesting chat after the talk about how they came to be a Perl shop and the resurgence of that language.
We're a Perl shop, which means nobody loves us.
Eric Bidelman

It is easy to take a simplistic view of the various software platforms that Google is working on right now. You might argue that building Chrome OS and Android are at cross purposes and that there is some confusion about which direction Google is moving in. Apps or web apps? Native code or portable code?
I had a long chat with Eric about the future of development, at Google and elsewhere, and got a very different impression. It is obvious that software is changing and that the focus is quickly moving away from the old desktop paradigms, towards the cloud and mobile. But this doesn't mean that desktop applications are becoming irrelavent, it just means they are going to be different. They are going to be more integrated with the cloud and they will run inside the browser, so we need modern browsers (and browser based operating systems) to host those applications. We will also need mobile platforms like iOS and Android to run native apps. Apps that are designed for, and take full advantage of, the hardware they are running on.
Gary Miller
I chatted with Gary about the future of spreadsheets, data visualization, GWT and Nick Lothian's awesome hacks.
Awesome
I talked to lots more people over the two days, but it is 2am, I am saying awesome way to much, have rambled on far more than I intended and really should go to bed. Goodnight! I am looking forward to seeing what is in store on Friday.
Permalink - Comments - Tags: Development,Google
Python vs Go Cage Match! Fight!
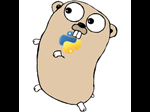
So this is not exactly a comparison between Python and Go. I just wanted to use my Gopher eating a Python logo image.
I love Python, it is sleeping in on Saturday mornings, my favorite book being picked up by HBO, baby holding onto pinky finger ... well, you get the idea, I like it a lot. I use it extensively for the piles and piles of code I write that never sees the light of day. Code that pre-processes files, scrapes web sites, munges together data sets, but doesn't actually end up anywhere near a user. When I saw Rob Pike talk about Go at a Sydney GTUG meeting, it occurred to me that a slick, modern, compiled language might be worth looking at for some of these behind the scenes projects that I embark upon.
Compiled vs Interpreted
Ok, duh! This is why I am here. I love Python with the heat of a thousand suns, but waiting a couple of hours for a process to finish, cools my adour a little. Compiled languages are fast and, from what I have seen, Go is a fast compiled language that also compiles fast. Fast is a feature. I have stuff to finish so I can go and watch The Sopranos.
Strong vs loose types
Occasionally, when I find myself in a particularly self deprecating frame of mind, I feel like I fit the mold of a duct tape programmer a little too much. Not in Joel Spolsky's generous, smart and get things done kind of way, but in the dumb and makes lots of mistakes kind of way. For me programming in a loosely typed language (like Python or PHP) tends to be a sequence of tiny, and sometimes humiliating, steps from a hideously broken thing to something approximating a working system. It would be nice if I could build something that was a little less flawed from the beginning. My experience is that a strongly typed language helps me do that:
// ahh ... compile error :P
var testStringMap = map[string] int {8:"eight"}
Useful built-in types
When I am forced to work in languages (and to be fair, this doesn't happen very often any more) that don't have strings, maps, arrays just built-in, it causes me physical pain:
STL I used to like you because it beat writing this stuff myself but, you kinda suck.
typedef std::map StringIntegerMap;
StringIntegerMap mapThatMakesMeTypeTooMuch;
int theNumberEight = 8;
std::string alsoTheNumberEight ("Eight");
mapThatMakesMeTypeTooMuch.insert(StringIntegerMap::value_type(theNumberEight, alsoTheNumberEight));
StringIntegerMap::const_iterator it;
it = mapThatMakesMeTypeTooMuch.find (theNumberEight);
if (it != mapThatMakesMeTypeTooMuch.end ())
std::string whatWasThatNumberAgain = it->second;
Python .. is awesome.
pythonDictionaryFTW = {8 : "Eight"}
theNumberEight = 8
if (theNumberEight in pythonDictionaryFTW):
whatWasThatNumberAgain = pythonDictionaryFTW[theNumberEight]
The map semantics in Go are just a little weird. I am sure I will get used to it. I got used to STL after all.
var testIntStringMap = map[int] string {8:"eight"}
var theNumberEight int = 8
if mappedString, ok := testIntStringMap[theNumberEight]; ok {
fmt.Printf ("Mapped String %s\n", mappedString)
}
Libraries
There are smarter, better looking programmers out there who can build awesome stuff that I want to use. I think the biggest reason not to switch from Python to Go is the range of third party libraries available for Python. Obviously this is something that will get resolved over time and I am sure there are lots of clever Google people squirreling away in their 20% time to build me awesome stuff (me personally). Beautiful Soup port for Go anyone?
Conclusions
I have lots more to learn about Go, but at this point I like it. It has a weird edgy feel about it that makes me feel a little smarter than I actually am, but it also reminds me a lot of Python and I luurve Python. I am hoping that I can find the time to actually build something real in the language and acquire enough knowledge to crush all resistance in the Go StackOverflow tag.
Permalink - Comments - Tags: Development,Google
Google Maps Sandbox
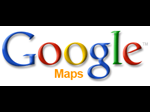
I have been a Google Maps enthusiast for some time and of late have been venting some of that enthusiasm in the google-maps tag on Stack Overflow. Despite having answered a bucket load of questions and done quite a bit of development with the Google Maps API, I sometimes encounter a question whose solution requires some empirical testing.
To facilitate these tests I set up a sandbox page on my site to host my cobbled together examples. The process has been quite rewarding and I have found myself delving into areas of the API which I would have ignored for my own projects. Here are a few of my favorites:
- Draw a square at an arbitrary point - This mostly involved taking someone else's awesome server side code, to determine a location an arbitrary distance/direction from a known point, and porting it to Javascript. Although I didn't get any questioner love on this one, the solution was still quite satisfying.
- Directions - Before this question I hadn't had a chance to play with the GDirections functionality in the API. This simple example just grabs the route between two points. The corresponding questioner was having some trouble getting this to work and it turned out the problem was unrelated.
- Travel time data - You can use the GDirections object without providing a map and directions div and just get the data back as a JSON response. In this demo I grabbed the driving time for the directions calculated between two points.
- Overlays - Had a lot of fun answering this question with a simple demo for using GTileLayerOverlay. The ability to leverage to the Google Maps API with your own tilesets is very powerful.
- Open street map tiles in Google maps - I implemented this demo for a question I didn't actually answer myself. I was browsing questions and found one that described how you can use the Google Maps API with an entirely separate map tile set, in this case from OpenStreetMap.org. This seemed like such a cool concept, I had to try it out.
Permalink - Comments - Tags: Development,Google
Tail Wagging Googlebot
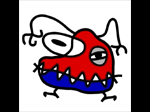
I discovered an interesting and somewhat elusive bug in my user validation stuff last night. With a little more sleep and some forethought I might have arrived at the solution a little earlier that I did, but I got there eventually.
I noticed, of the handfall of users that have signed up for my Timeline Project, not all had validated their accounts. I wondered about the reason why and decided to do a bit of testing. I follow a pretty standard model for account validation:
- User enters their new details.
- I add the details to the database and generate a random key.
- I send an email to the specified address with a URL back passing the key as a param.
- User clicks on the URL in the email and I match it to the database key I stored earlier. Then I erase the key in the user's database entry. I only need to do this once and I use the key for other stuff (password resets).
- If everything matches up, I set a valid flag on the user account.
So pretty straightforward? When I originally wrote the code, I tested with a couple of accounts, assumed everything was fine and moved on. I assumed wrong.
Re-testing this stuff, I created a whole pile of accounts and checked that they could be successfully validated. To my chagrin, I found that when I clicked on the validation URL, for a handful of them, I generated an error message saying that the key didn't match. This was something of a gumption trap, a random intermittent fail with a system that should be trivial.
I fell back on that mainstay of programming, debug writes. I already have a decent logging system, so I added a few more log messages when the validation URL gets fired. The results I found were puzzling to say the least:

For this log example, the validation succeeded (validate_pass), but the message immediately after it was extremely suspicious. An attempt to validate that user id from some obscure IP address that I hadn't seen in my logs before. My "it is always your fault" programmer instincts failed me and I jumped from one irrational and outlandish conclusion to the next. Eventually I came to my senses and decided to do a traceroute on the IP address:
When my activation email arrived in my Gmail inbox, that enthusiastic, tongue lolling, tail wagging Labrador of a GoogleBot immediately jumped on my validation URL and bounded off to get it. So if the GoogleBot hit the URL first, then the key would get reset and the subsequent real user request would fail. It is pretty satisfying when a classic intermittent and quite random race condition has a very simple solution. "It is ALWAYS your fault". I gotta remember that one.
Adding a few lines to my robots.txt file, which up until that point had been empty, ensured that the GoogleBot would refrain from hitting those fragile URLs. Bug Fixed.
Permalink - Comments - Tags: Development,Google
Google Adwords, Small Things amuse..
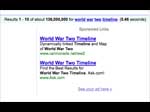
Thanks to Google and their Stimulus Offer I have seventy bucks of adwords to spend. I am not sure why, but I got a kick out of seeing my ad in sponsored search results. It is probably just a combination of 1am and codeine for back pain.
Permalink - Comments - Tags: Development,World War Two,Google
[First Page] [Prev] Showing page 4 of 4 pages [Next] [Last Page]